准备工作
事先准备好一个E:\news.txt文件,内容如下,总字节数为11
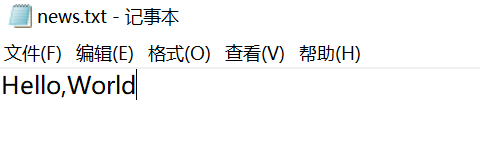
常用构造器
-
public FileInputStream(String name) throws FileNotFoundException
传入形参为String类型,表示文件的路径
-
public FileInputStream(File file) throws FileNotFoundException
传入形参为File对象,表示文件绑定的File对象
1 2 3 4 5
|
FileInputStream fileInputStream1 = new FileInputStream("E:\\news.txt");
FileInputStream fileInputStream2 = new FileInputStream(new File("E:\\news.txt"));
|
available
用于获取文件的总字节数
public native int available() throws IOException
1 2 3 4
| FileInputStream fileInputStream = new FileInputStream(new File("E:\\news.txt")); System.out.println(fileInputStream.available());
|
read
从文件中读取数据,当文件不存在时会抛出异常FileNotFoundException
有三种重载形式
-
public int read() throws IOException
一个字节一个字节读取文件数据,返回的是读取到的字节,达到文件末尾时返回-1
-
public int read(byte b[]) throws IOException
每次按照字节数组b的大小来读取字节,将读取的字节存到字节数组b中,返回读取的字节数,达到文件末尾时返回-1
-
public int read(byte b[], int off, int len) throws IOException
每次按照len的大小来读取字节,将读取的字节存到字节数组b中,从off位置开始存,返回读取的字节数,达到文件末尾时返回-1
注意应该满足len+off<=b.length
,否则会抛出异常IndexOutOfBoundsException
1 2 3 4 5 6 7 8 9 10
| FileInputStream fileInputStream = new FileInputStream(new File("E:\\news.txt"));
int readData = 0; while ((readData = (fileInputStream.read())) != -1) { System.out.print((char)readData); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
|
byte[] b = new byte[5]; int readLen = 0; while ((readLen = fileInputStream.read(b)) != -1) { String result = new String(b, 0, readLen); System.out.println("readLen = " + readLen + "," + "result = " + result); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
byte[] b = new byte[5]; int readLen = 0;
while ((readLen = fileInputStream.read(b, 0, 5)) != -1) { String result = new String(b, 0, readLen); System.out.println("readLen = " + readLen + "," + "result = " + result); }
|
close
用于关闭FileInputStream流
public void close() throws IOException
养成好习惯,输入输出流用完即关
1 2 3
| FileInputStream fileInputStream = new FileInputStream(new File("E:\\news.txt"));
fileInputStream.close();
|
常用构造器
-
public FileOutputStream(String name) throws FileNotFoundException
通过String文件路径来构造FileOutputStream对象,默认为覆盖
-
public FileOutputStream(String name, boolean append) throws FileNotFoundException
通过String文件路径来构造FileOutputStream对象,append为true表示追加,为false表示覆盖
-
public FileOutputStream(File file) throws FileNotFoundException
通过File对象来构造FileOutputStream对象,默认为覆盖
-
public FileOutputStream(File file, boolean append) throws FileNotFoundException
通过File文件路径来构造FileOutputStream对象,append为true表示追加,为false表示覆盖
1 2 3
| String fileName = "E:\\news.txt"; FileOutputStream fileOutputStream = new FileOutputStream(fileName);
|
1 2 3 4
| String fileName = "E:\\news.txt";
FileOutputStream fileOutputStream = new FileOutputStream(fileName, true);
|
1 2 3
| File file = new File("E:\\news.txt"); FileOutputStream fileOutputStream = new FileOutputStream(file);
|
1 2 3 4
| File file = new File("E:\\news.txt");
FileOutputStream fileOutputStream = new FileOutputStream(file, true);
|
flush
一般情况下写入数据到文件中会先把文件写入到缓冲区,待缓冲区满后再将数据写入到文件中,由于存在缓冲区不满的情况,就有可能出现数据部分不写入文件中的情况,需要强制将缓存区的数据写入文件中,这就是flush的作用
public void flush() throws IOException
养成好的习惯,使用输出流的时候及时调用flush
1 2 3 4
| File file = new File("E:\\news.txt"); FileOutputStream fileOutputStream = new FileOutputStream(file);
fileOutputStream.flush();
|
close
用于关闭FileInputStream
public void close() throws IOException
1 2 3 4
| File file = new File("E:\\news.txt"); FileOutputStream fileOutputStream = new FileOutputStream(file);
fileOutputStream.close();
|
write
写入数据到文件中,当文件不存在时会自动创建文件
-
public abstract void write(int b) throws IOException
写入字节b到文件中
-
public void write(byte b[]) throws IOException
写入字节数组到文件中
-
public void write(byte b[], int off, int len) throws IOException
写入字节数组从off开始的长度为len的数据到文件中
重载1
1 2 3 4 5 6 7
| File file = new File("E:\\news.txt"); FileOutputStream fileOutputStream = new FileOutputStream(file);
fileOutputStream.write(67); fileOutputStream.flush(); fileOutputStream.close();
|
结果
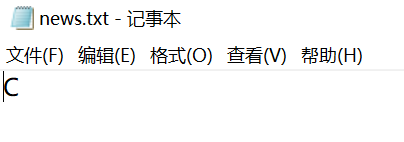
重载2
1 2 3 4 5 6 7 8 9 10
| File file = new File("E:\\news.txt"); FileOutputStream fileOutputStream = new FileOutputStream(file);
String outputData = "Hello,World"; byte[] outputDataToBytes = outputData.getBytes();
fileOutputStream.write(outputDataToBytes); fileOutputStream.flush(); fileOutputStream.close();
|
结果
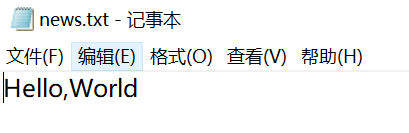
重载3
1 2 3 4 5 6 7 8 9 10
| File file = new File("E:\\news.txt"); FileOutputStream fileOutputStream = new FileOutputStream(file);
String outputData = "Hello,World"; byte[] outputDataToBytes = outputData.getBytes();
fileOutputStream.write(outputDataToBytes, 2, 3); fileOutputStream.flush(); fileOutputStream.close();
|
结果